Async/Await: A Beginner's Guide
- Alexey Parkhomenko
- 4 апр. 2023 г.
- 3 мин. чтения
Async/Await is a modern approach to handling asynchronous tasks in Swift, introduced in Swift 5.5. This powerful feature makes it easier for developers to write clean, concise, and readable code when working with asynchronous operations. In this blog post, we'll explore how to use Async/Await in iOS development, with code examples to help you get started.
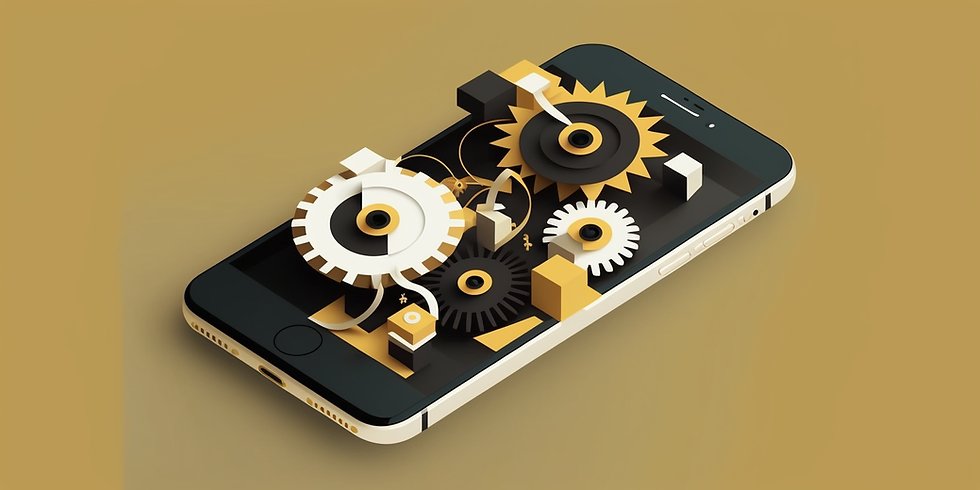
Table of Contents:
Understanding Async/Await
Getting Started with Async/Await
Using Task and TaskGroup
Error Handling with Async/Await
AsyncImage in SwiftUI
Understanding Async/Await
Async/Await is a syntactic sugar that simplifies the process of writing asynchronous code. It allows you to write code that appears to be synchronous, even though it's executing asynchronously. This helps to reduce the complexity of callback-based code, resulting in code that's easier to read and maintain.
Getting Started with Async/Await
To demonstrate the use of Async/Await, let's create a simple function that simulates downloading a JSON file from a URL.
First, add the following 'downloadJSON' function:
func downloadJSON(from url: URL) async throws -> Data {
let (data, _) = try await URLSession.shared.data(from: url)
return data
}
Now, let's create an async function to fetch and decode the JSON data:
struct User: Codable {
let id: Int
let name: String
}
func fetchUser() async throws -> User {
let url = URL(string: "https://api.example.com/user")!
let data = try await downloadJSON(from: url)
let decoder = JSONDecoder()
let user = try decoder.decode(User.self, from: data)
return user
}
To call the 'fetchUser' function from an async context, use the 'async' keyword:
@MainActor
func displayUser() async {
do {
let user = try await fetchUser()
print("User: \(user.name)")
} catch {
print("Error: \(error.localizedDescription)")
}
}
Using Task and TaskGroup
'Task' and 'TaskGroup' are structures in Swift Concurrency that facilitate the execution of multiple asynchronous tasks concurrently. By employing these tools, you can optimize your app's performance and responsiveness when handling several operations at once.
Let's demonstrate how to fetch multiple users concurrently with the help of Task and TaskGroup:
func fetchUsers(userIds: [Int]) async throws -> [User] {
try await withThrowingTaskGroup(of: User.self) { taskGroup in
for userId in userIds {
let url = URL(string: "https://api.example.com/user/\(userId)")!
taskGroup.addTask {
let data = try await downloadJSON(from: url)
let decoder = JSONDecoder()
let user = try decoder.decode(User.self, from: data)
return user
}
}
var users: [User] = []
for try await user in taskGroup {
users.append(user)
}
return users
}
}
In this example, we use 'withThrowingTaskGroup(of:_:)' to create a new task group, allowing us to perform multiple asynchronous tasks concurrently. Tasks are added to the group using 'taskGroup.addTask'. We then collect the results by iterating over the tasks in the group using a for-await loop.
Error Handling with Async/Await
Handling errors with async functions is similar to handling errors in synchronous code. You can use do-catch statements to catch errors thrown by async functions.
@MainActor
func displayUsers() async {
do {
let userIds = [1, 2, 3, 4, 5]
let users = try await fetchUsers(userIds: userIds)
for user in users {
print("User: \(user.name)")
}
} catch {
print("Error: \(error.localizedDescription)")
}
}
In this example, we fetch multiple users concurrently using the 'fetchUsers' function. If any error occurs during the fetching process, it will be caught by the do-catch statement, allowing us to gracefully handle the error and inform the user, if necessary.
AsyncImage in SwiftUI
SwiftUI introduces 'AsyncImage' for asynchronous image loading, which simplifies the process of loading and displaying remote images. 'AsyncImage' can be used with Async/Await to make image loading even more straightforward.
Here's an example of using 'AsyncImage' in a SwiftUI view:
import SwiftUI
struct UserView: View {
let imageUrl: URL
var body: some View {
AsyncImage(url: imageUrl) { phase in
switch phase {
case .empty:
ProgressView()
case .success(let image):
image.resizable().scaledToFit()
case .failure:
Image(systemName: "photo.fill")
.foregroundColor(.red)
@unknown default:
fatalError("Unknown AsyncImagePhase")
}
}
}
}
Conclusion
Async/Await is a powerful feature in Swift that simplifies asynchronous programming. It allows developers to write clean, readable, and maintainable code when working with asynchronous tasks. In this blog post, we've covered the basics of using Async/Await in iOS development, including error handling, Task and TaskGroup, and AsyncImage in SwiftUI. With these tools in your arsenal, you'll be able to create more efficient and user-friendly apps that handle asynchronous tasks with ease.
Comments